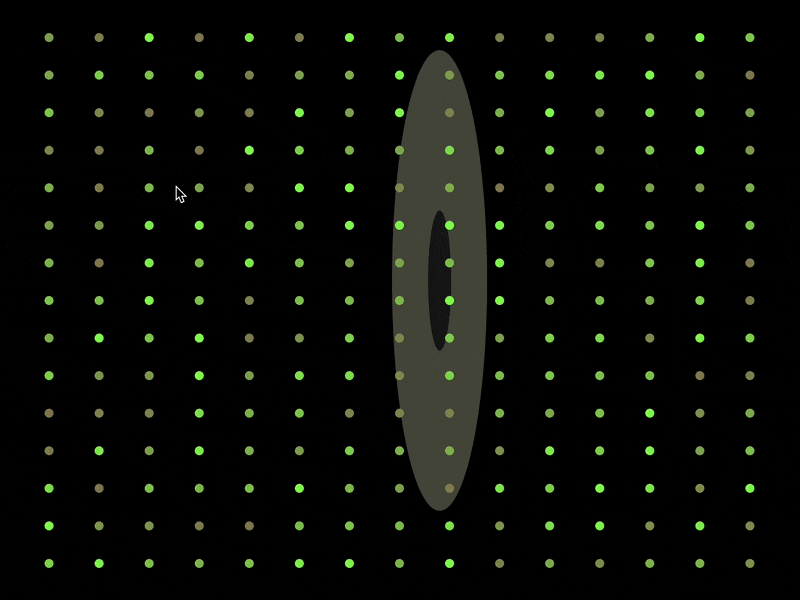
Project Brief
Create a sketch that would simulate an existing natural system - look at physics, biology, and other natural sciences for good examples. Start with the environment - where is the system situated? What are the forces the environment might exert on the system? Examine the agents in the system, their relationships to each other and the environment they are in. The look at how this system would develop over time. What are the rules that you are going to come up with, what are the parameters you are going to feed into them and what effect will the changes have on the development of the system.
∙ Create classes of entities to represent the components of the system
∙ Use vectors to represent forces existing in the system
∙ Use randomness or noise to generate at least one
∙ Add direct or indirect mouse or keyboard controls
∙ Use vectors to represent forces existing in the system
∙ Use randomness or noise to generate at least one
∙ Add direct or indirect mouse or keyboard controls
Experimenting Using Classes in p5.js
Using knowledge from previous workshops in the last two weeks of October, I developed the idea of having a meteor shower appear as a person's mouse moves in. I looked at the class we had created called Particles.js where we had given the characteristics such as movement, acceleration, and position.
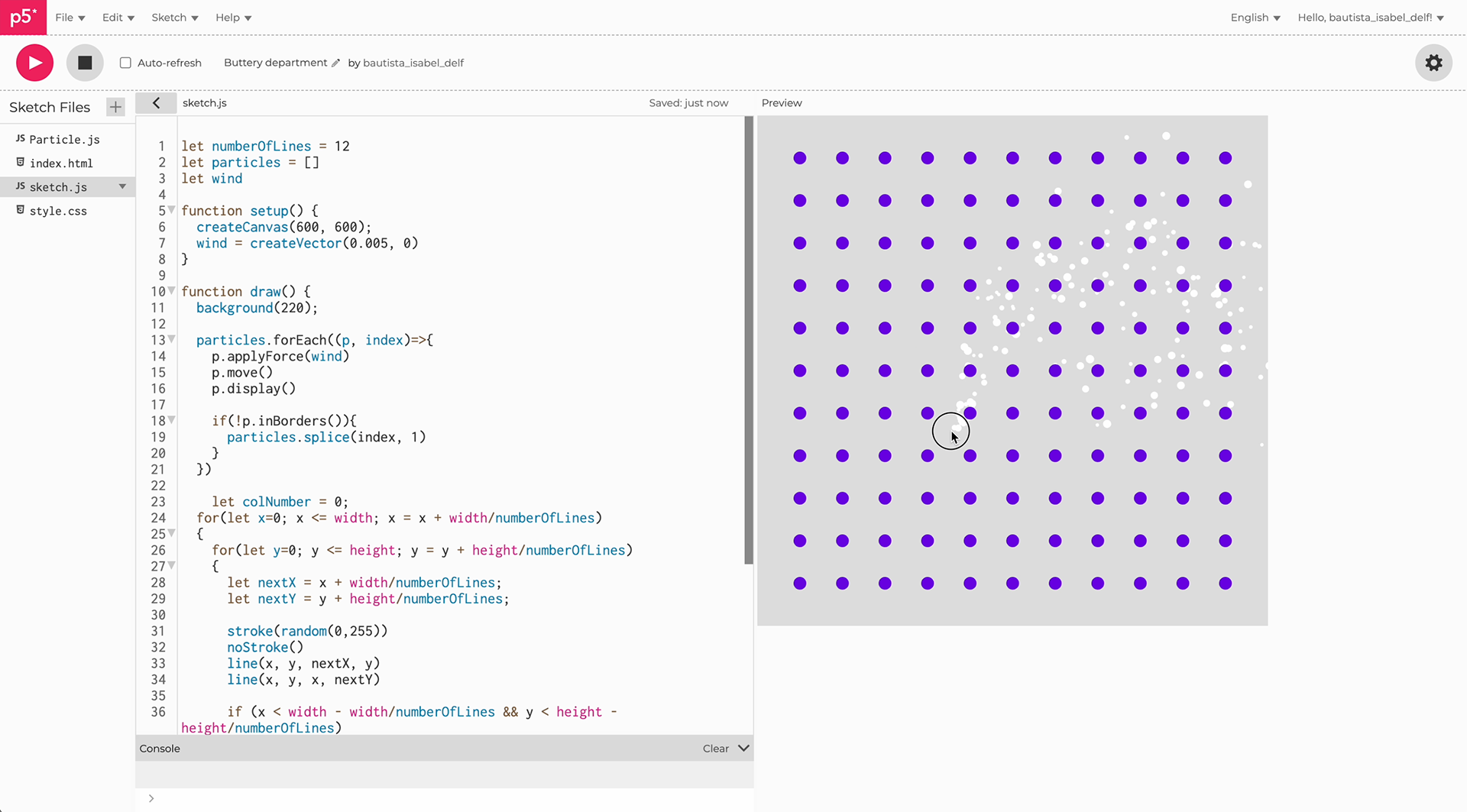
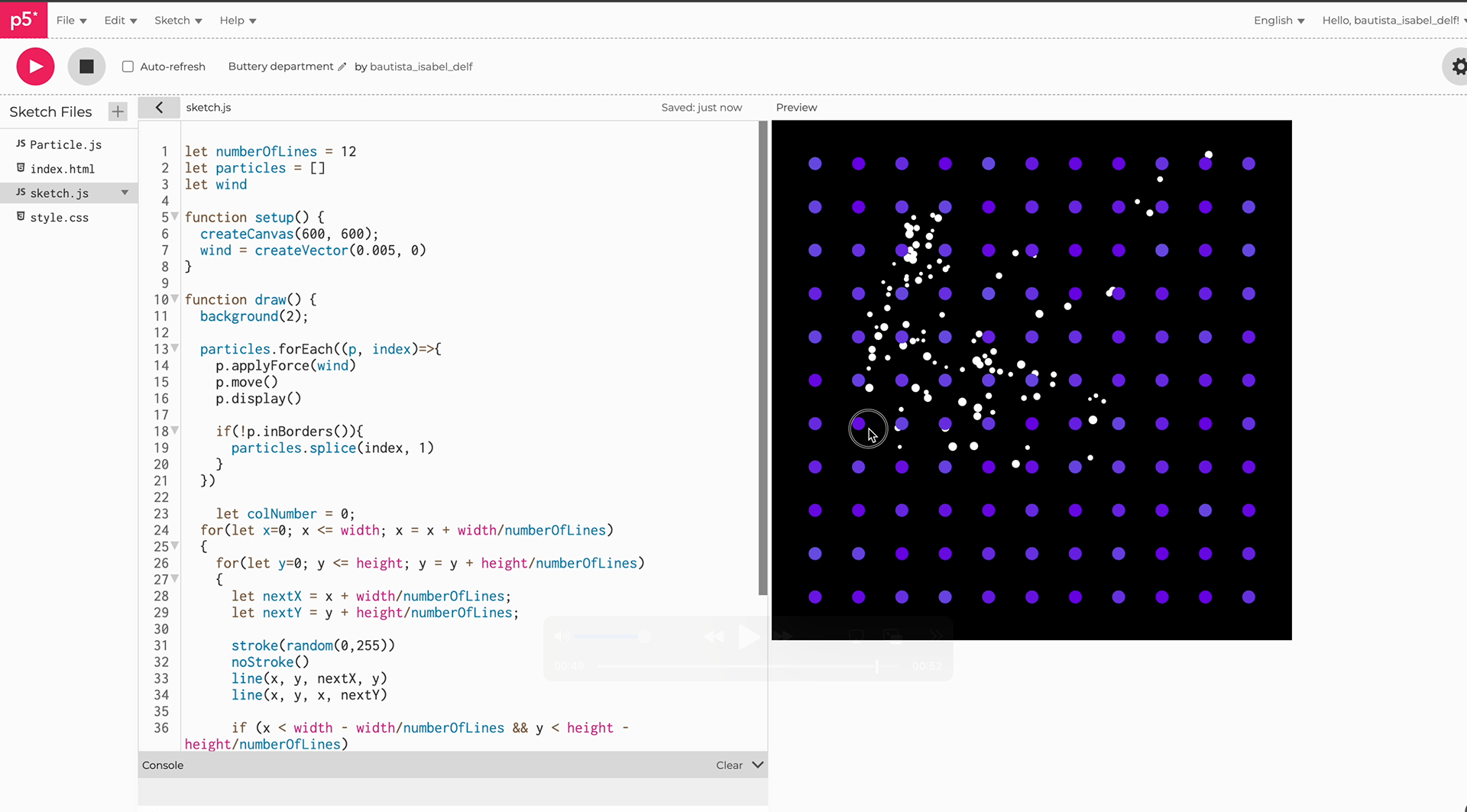
I reused the code I had developed in a previous project where I had the background of gridded circles rotating along the axis and intended to create the same in this project. Although the rotating circles would have been a nice addition, as a designer I needed to think about reducing distractions to ensure cohesion in the design and only incorporate the elements necessary to execute.
The next steps: incorporating a rotating planet and the placement of the ellipse. The video below shows my trial-and-error process while experimenting with the placement and color of these elements.
My thought process going through this project (video has no audio)
After making the elements I needed, I was trying to figure out how to make the particles become attracted to the ellipse and move towards it no matter where it is placed.
The Winds of Change
I will admit that knowing what needs to change as a designer is second nature, but knowing where the change needs to happen when looking at code takes time. I knew that the movement of the particle was the main component I needed to look at, so in going back and forth between the constructor and the sketch file, I was looking to find the areas that needed to be modified.
Another factor that played into this was knowing how to write the correct code, so I reached out to my tutor for help to walk me through this. Since I had the particles move in a more natural manner as if blown by wind, then I would need to actually make it so that the particles specifically move in a direct manner, or rather, be pulled in the direction of the ellipse.
My tutor and I had more of a conversation as we went through the changes. This helps me retain the information about what I am doing as I code while also learning how to talk about my work outside of my designer role.
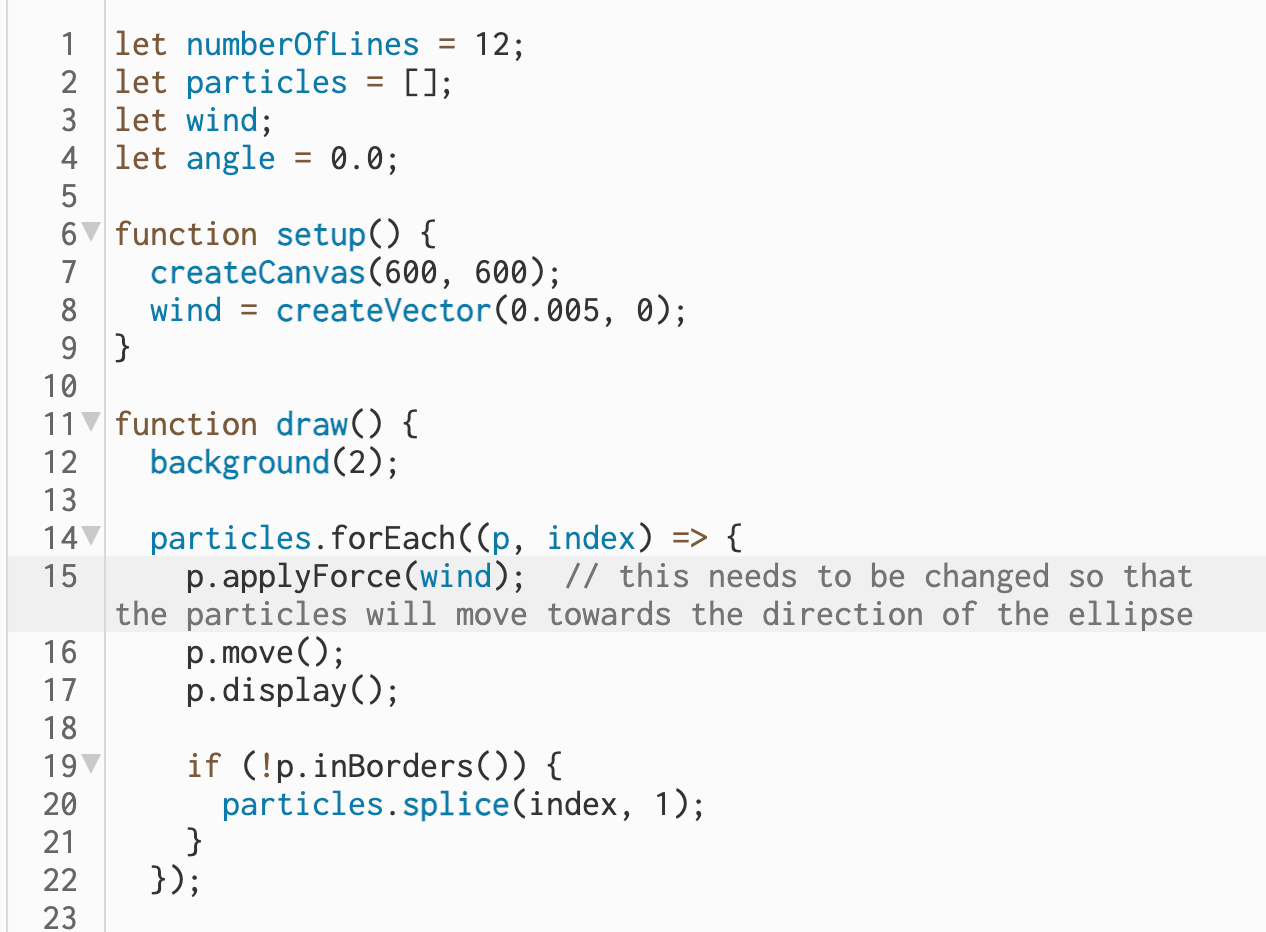
The Final Interactions
After going through the particles class and the sketch, I had finally completed my project! Below is the video that takes you through after I've made the changes and what the interactions look like. *Video has no audio*
Click and drag on the canvas to produce small meteors in space! The meteors will become attracted to the lovely black hole and remain within its force.
let numberOfLines = 16;
let particles = [];
let particles = [];
let blackHole;
let angle = 65.75;
let angle = 65.75;
function setup() {
createCanvas(800, 600);
blackHole = createVector(440, 280);
}
createCanvas(800, 600);
blackHole = createVector(440, 280);
}
function draw() {
background(2);
background(2);
particles.forEach((p, index) => {
let pullDirection = p5.Vector.sub(blackHole, p.position).normalize();
p.applyForce(pullDirection);
p.move();
p.display();
let pullDirection = p5.Vector.sub(blackHole, p.position).normalize();
p.applyForce(pullDirection);
p.move();
p.display();
if (!p.inBorders()) {
particles.splice(index, 1);
}
});
particles.splice(index, 1);
}
});
//ellipses will be the "black hole"
fill(77, 79, 66);
ellipse(blackHole.x, blackHole.y, 95, 460);
noStroke();
fill(77, 79, 66);
ellipse(blackHole.x, blackHole.y, 95, 460);
noStroke();
//center of "black hole"
fill(23, 23, 22);
ellipse(440, 280, 23, 140);
fill(23, 23, 22);
ellipse(440, 280, 23, 140);
let colNumber = 0;
for (let x = 0; x <= width; x = x + width / numberOfLines) {
for (let y = 0; y <= height; y = y + height / numberOfLines) {
let nextX = x + width / numberOfLines;
let nextY = y + height / numberOfLines;
for (let x = 0; x <= width; x = x + width / numberOfLines) {
for (let y = 0; y <= height; y = y + height / numberOfLines) {
let nextX = x + width / numberOfLines;
let nextY = y + height / numberOfLines;
noStroke();
line(x, y, nextX, y);
line(x, y, x, nextY);
line(x, y, nextX, y);
line(x, y, x, nextY);
if (
x < width - width / numberOfLines &&
y < height - height / numberOfLines
) {
fill(138, random(128, 255), 87);
noStroke();
circle(nextX, nextY, 9);
}
}
}
x < width - width / numberOfLines &&
y < height - height / numberOfLines
) {
fill(138, random(128, 255), 87);
noStroke();
circle(nextX, nextY, 9);
}
}
}
//planet rotatation
rotate(angle);
translate(10, -160);
drawPlanet();
angle = angle + 0.005;
}
rotate(angle);
translate(10, -160);
drawPlanet();
angle = angle + 0.005;
}
function drawPlanet() {
noStroke();
fill(25, 100, 200);
circle(width / 4, height / 3, 100);
}
noStroke();
fill(25, 100, 200);
circle(width / 4, height / 3, 100);
}
function mouseDragged() {
particles.push(new Particle(mouseX, mouseY));
}
particles.push(new Particle(mouseX, mouseY));
}