Project Brief
The goal of this project is to create an animation that depicts a timekeeping system designed for an alien civilization. The clock cannot display time tied to the rotation of the Earth - years, months, days, hours, minutes, etc. There is freedom to invent a system of time - however large or small. If the clock is no longer tied to celestial movement - how else can the passing of time be expressed? Would the time be continuous or discrete? Cyclical or unidirectional? How will time be divided into sub-elements and what visual tools would be used to represent distinctions: color, shape, size, order?
Technical Requirements:
∙ Use arrays and loops
∙ Use rotation with the transformation matrix
∙ Use either trigonometric functions or intervals (or both if need be)
∙ Use rotation with the transformation matrix
∙ Use either trigonometric functions or intervals (or both if need be)
Assuming Aliens Can Breathe
The way I thought about time revolved around how things begin and end as well as things needing to be constant. I thought about the rings of a tree which led my mind into thinking about biodomes and how self-sustaining they are. Thus, the emergence of breathing had my thoughts swirling around the idea of looking at time based on that function alone. Breathing varies being-to-being, so why not let time become an independent construct?
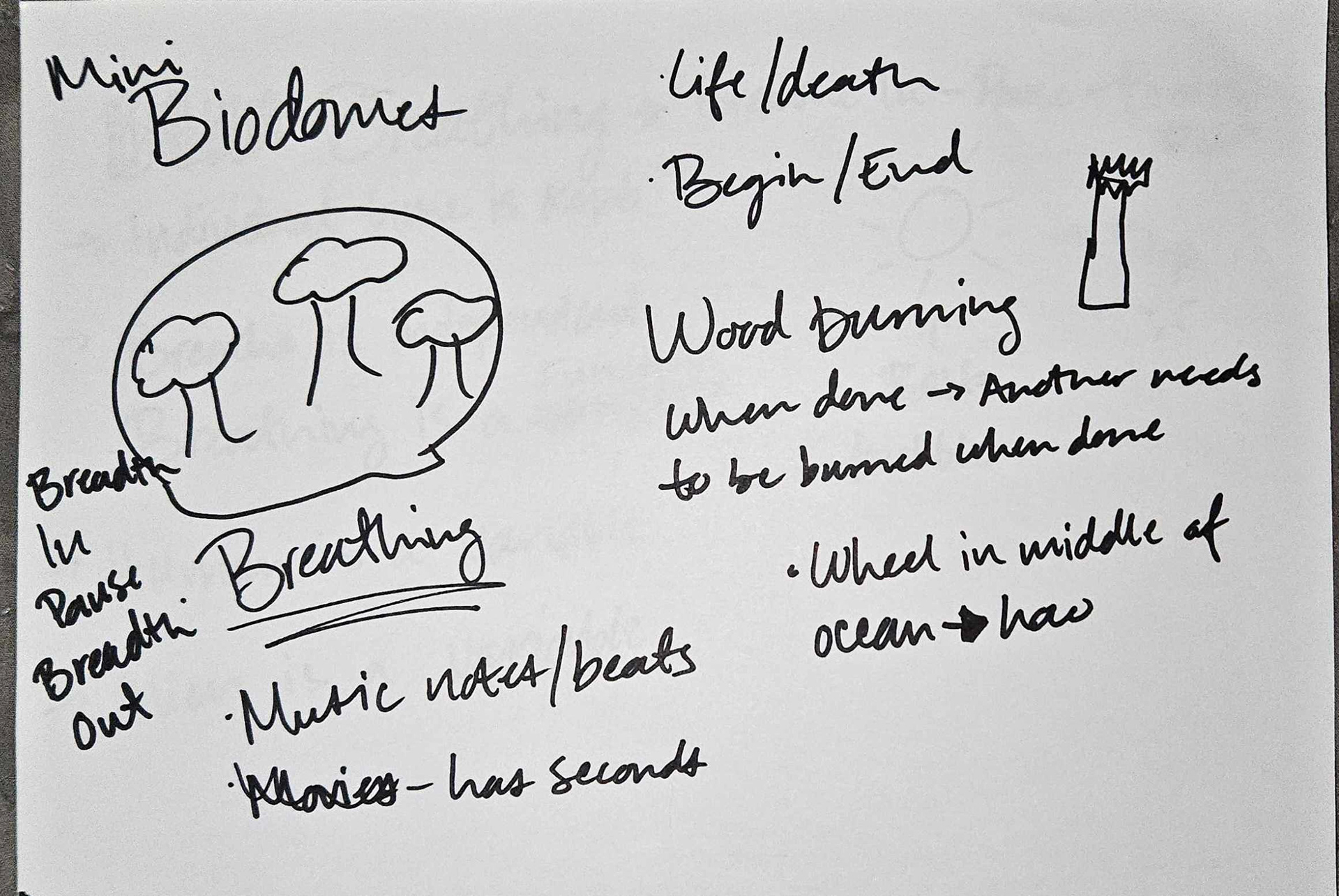
Brainstorming ideas not related to how we currently track time
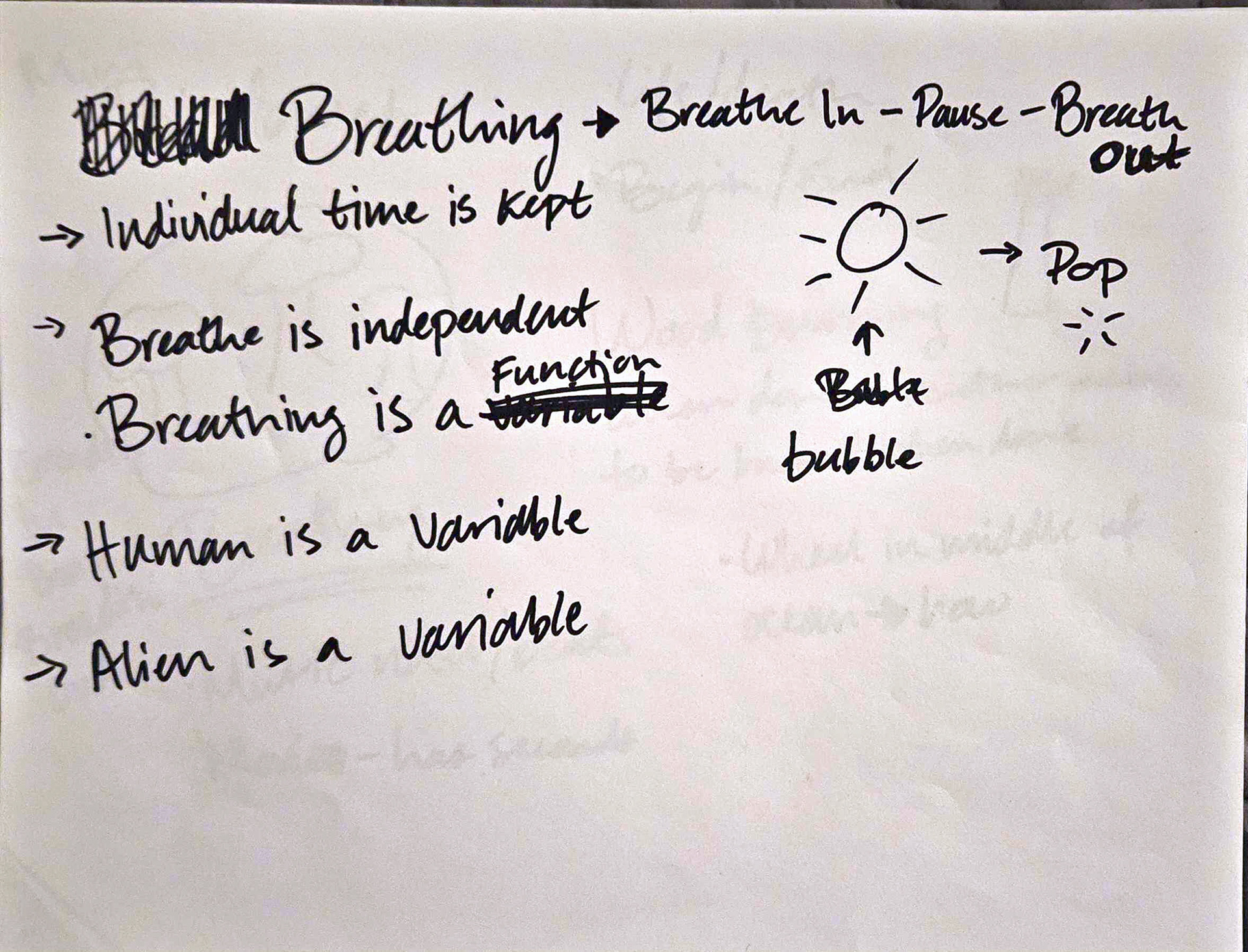
Break down of what my variables and functions would look like for my code
Once I figured out how the alien civilization could track time, I started writing down what my potential variables and functions could look like before I stepped into the coding environment of p5.js. Writing down the variables and breaking down the functions of breathing are what help me understand the steps I need to account for so that I don't lose myself in coding linguistics.
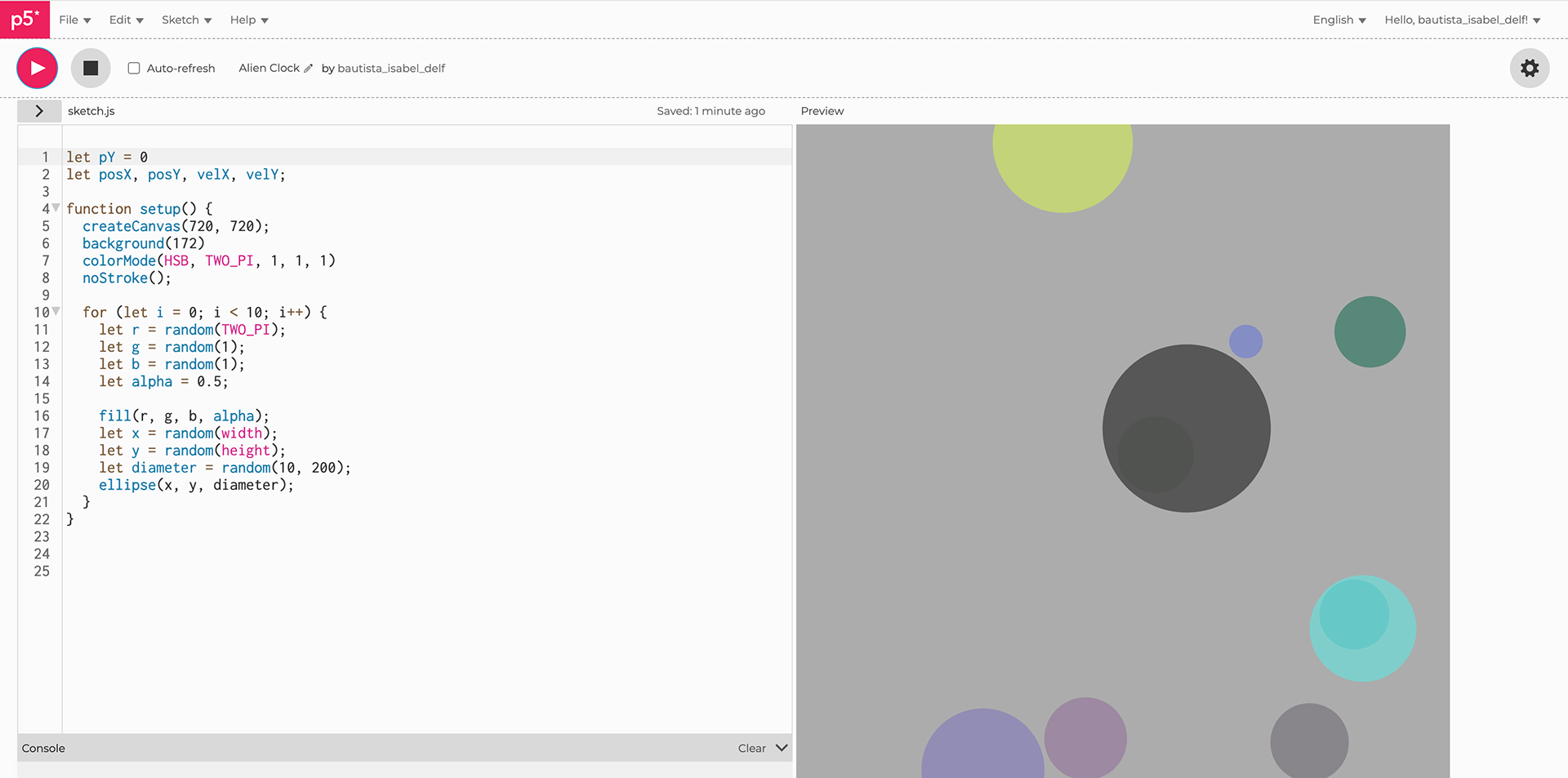
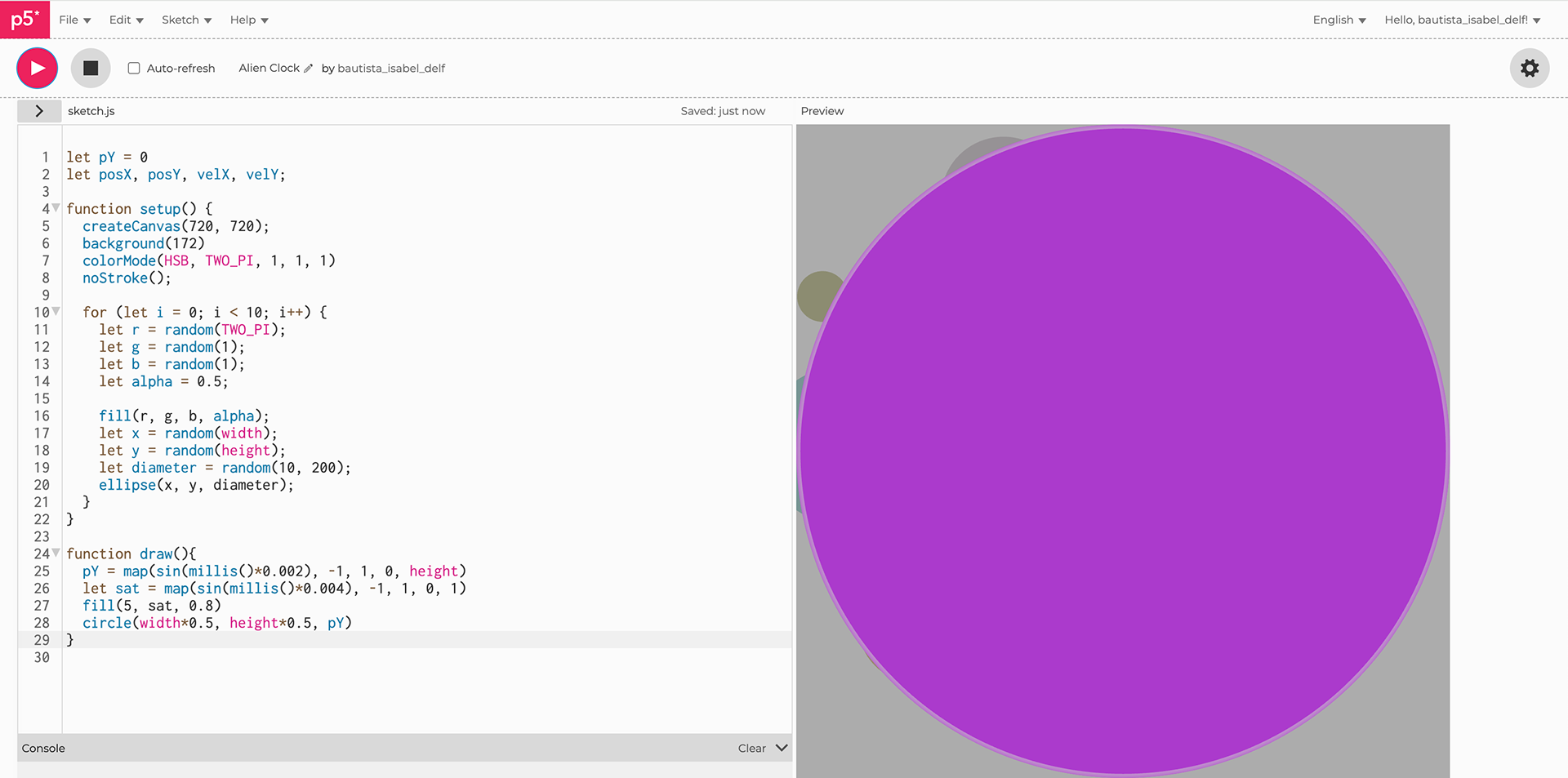
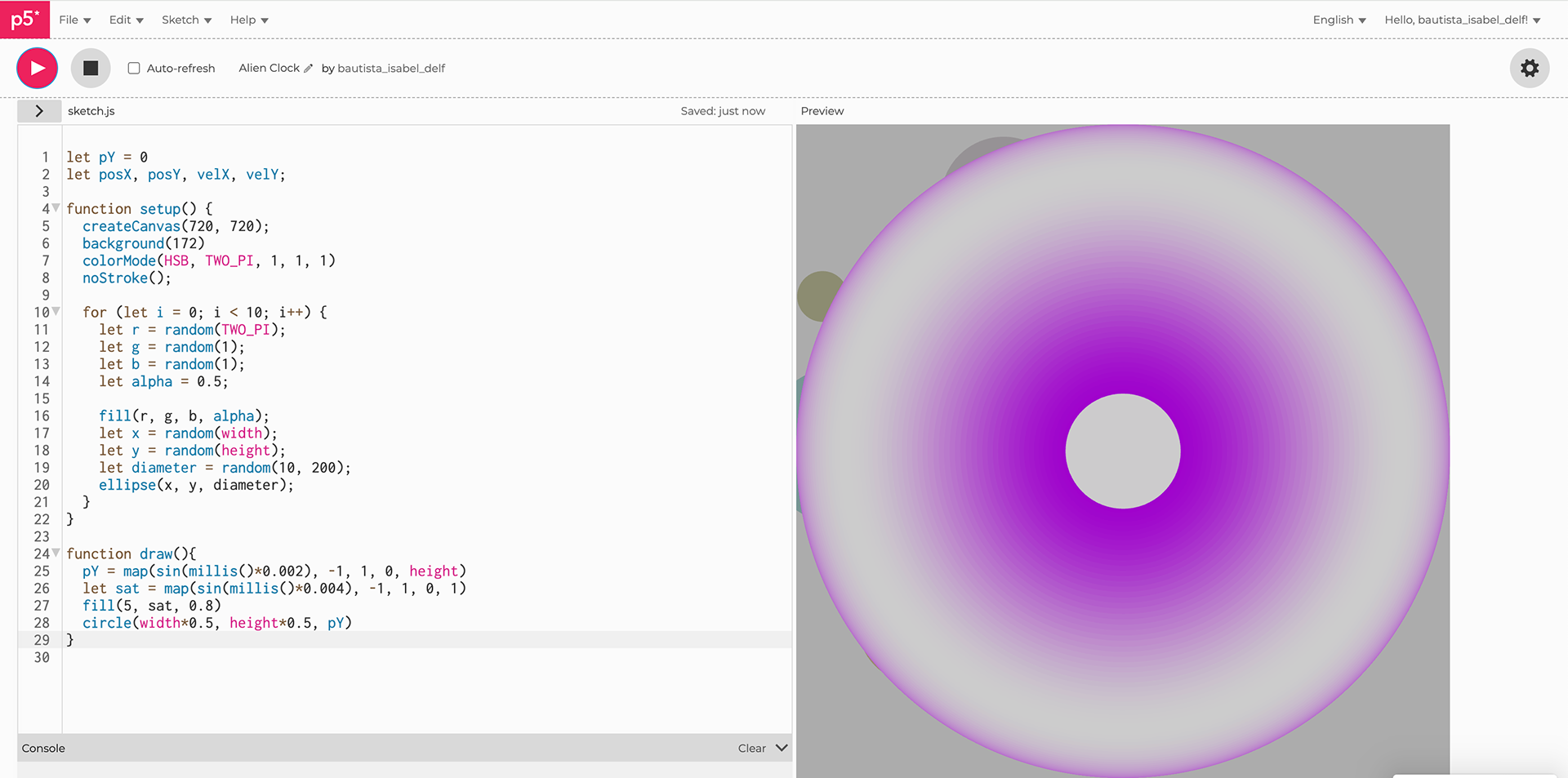
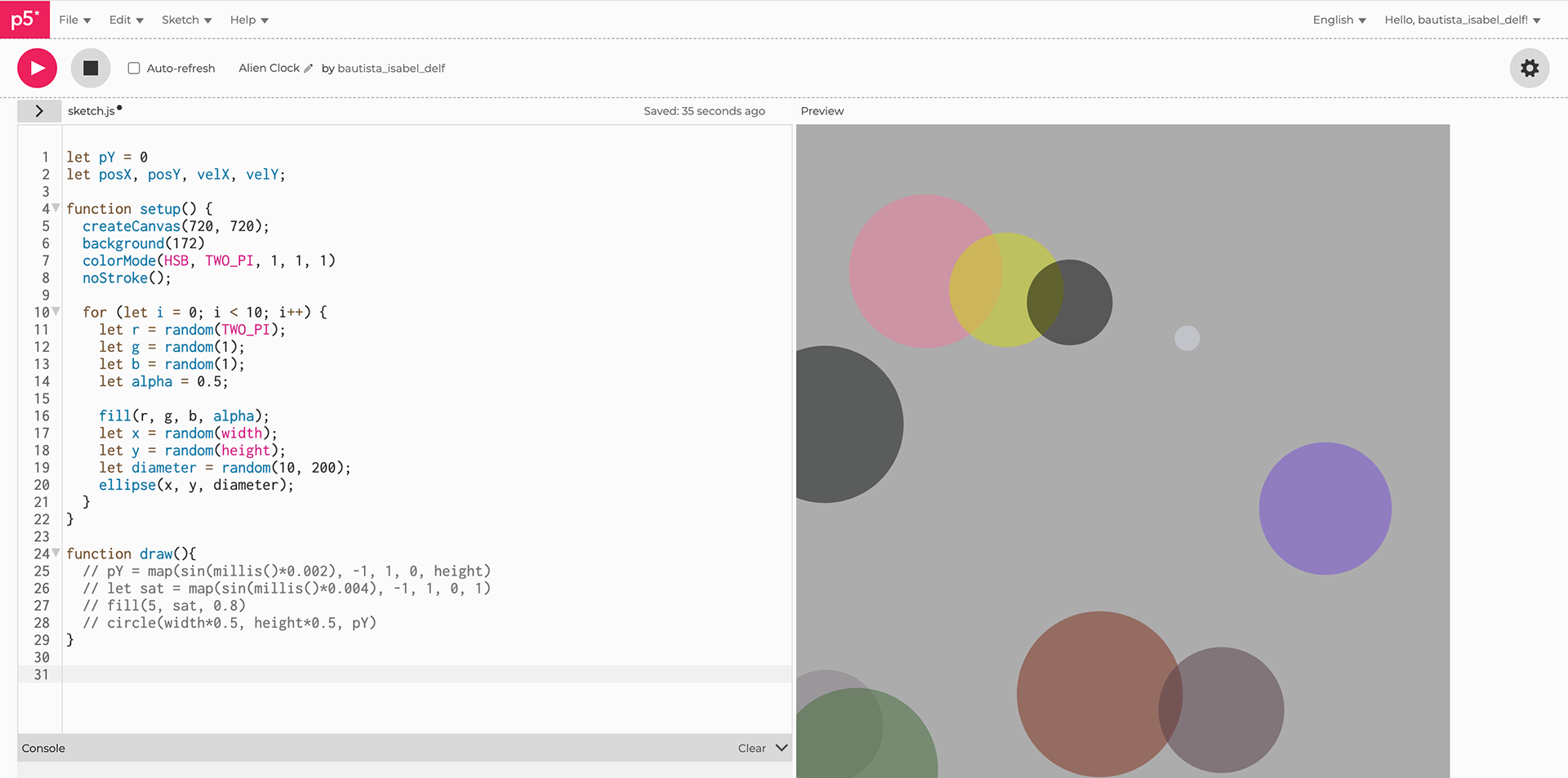
The Alien Clocks
After spending some time experimenting and documenting the before and after results, it was time to move on into the next phase of the clock.
Below the video is a copy of my code - the before and after. Feel free to experiment with this and have fun!
let pY = 0
// let posX, posY, velX, velY;
// let posX, posY, velX, velY;
function setup() {
createCanvas(720, 720);
background(172)
colorMode(HSB, TWO_PI, 1, 1, 1)
noStroke();
for (let i = 0; i < 10; i++) {
let r = random(TWO_PI);
let g = random(1);
let b = random(1);
let alpha = 0.5;
createCanvas(720, 720);
background(172)
colorMode(HSB, TWO_PI, 1, 1, 1)
noStroke();
for (let i = 0; i < 10; i++) {
let r = random(TWO_PI);
let g = random(1);
let b = random(1);
let alpha = 0.5;
fill(r, g, b, alpha);
let x = random(width);
let y = random(height);
let diameter = random(10, 200);
ellipse(x, y, diameter);
}
}
let x = random(width);
let y = random(height);
let diameter = random(10, 200);
ellipse(x, y, diameter);
}
}
function draw(){
pY = map(sin(millis()*0.002), -1, 1, 0, height)
let sat = map(sin(millis()*0.004), -1, 1, 0, 1)
fill(5, sat, 0.8)
circle(width*0.5, height*0.5, pY)
pY = map(sin(millis()*0.002), -1, 1, 0, height)
let sat = map(sin(millis()*0.004), -1, 1, 0, 1)
fill(5, sat, 0.8)
circle(width*0.5, height*0.5, pY)
}
let pY = 0
let numCircles = 12;
const circleX = [];
const circleY = [];
const circleColor = [];
const circlePulse = [];
let numCircles = 12;
const circleX = [];
const circleY = [];
const circleColor = [];
const circlePulse = [];
function setup() {
createCanvas(720, 720);
background(172)
colorMode(HSB, TWO_PI, 1, 1, 1)
noStroke();
for (let i = 0; i < 14; i++) {
let r = random(TWO_PI);
let g = random(1);
let b = random(8);
let alpha = 0.5;
fill(r, g, b, alpha);
let x = random(width);
let y = random(height);
let diameter = random(10, 450);
ellipse(x, y, diameter);
}
for(let i = 0; i < numCircles; i++) {
circleX[i] = random(width);
circleY[i] = random(height);
circleColor[i] = random();
circlePulse[i] = random(0.001, 0.006)
}
}
createCanvas(720, 720);
background(172)
colorMode(HSB, TWO_PI, 1, 1, 1)
noStroke();
for (let i = 0; i < 14; i++) {
let r = random(TWO_PI);
let g = random(1);
let b = random(8);
let alpha = 0.5;
fill(r, g, b, alpha);
let x = random(width);
let y = random(height);
let diameter = random(10, 450);
ellipse(x, y, diameter);
}
for(let i = 0; i < numCircles; i++) {
circleX[i] = random(width);
circleY[i] = random(height);
circleColor[i] = random();
circlePulse[i] = random(0.001, 0.006)
}
}
function draw(){
for (let i = 0; i < numCircles; i++) {
pY = map(sin(millis()*circlePulse[i]), -1, 1, 0, 95)
let sat = map(sin(millis()*0.004), -1, 1, 0, 1)
fill(circleColor[i], sat, 0.9)
circle(circleX[i], circleY[i], pY)
}
}
for (let i = 0; i < numCircles; i++) {
pY = map(sin(millis()*circlePulse[i]), -1, 1, 0, 95)
let sat = map(sin(millis()*0.004), -1, 1, 0, 1)
fill(circleColor[i], sat, 0.9)
circle(circleX[i], circleY[i], pY)
}
}